Learn Multithreading With Modern C++
- 06.08.2022
- 83
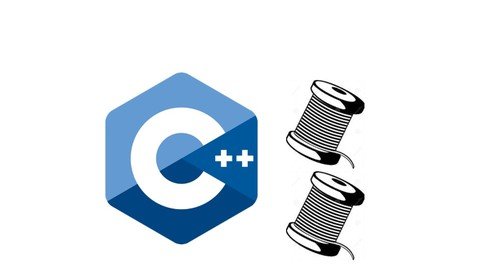
Last updated 7/2022
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 1.80 GB | Duration: 7h 0m
Learn how to write portable threaded C++ code which unleashes the power of modern multi-processor hardware!
What you'll learn
How to write portable multi-threaded code in C++
Basic principles of multi-threading which will be applicable in all languages
Understanding of concurrency
Knowledge of C++17 parallel algorithms
Requirements
Familiarity with Advanced Modern C++ features such as lambda expressions and move semantics
Access to C++11 compiler (C++14 preferred.) Parallel algorithms require C++17
Intended for students who have taken my courses "Learn Advanced Modern C++" or "Update to Modern C++"
Proficiency in English (B2 level, preferably C1)
Description
Multithreading is used in many areas of computing, including graphics processing, machine learning and Internet stores. An understanding of threads is essential to make full use of the capabilities of modern hardware.C++ now provides direct support for threads, making it possible to write portable multithreaded programs which have well-defined behaviour, without requiring any external libraries.This course thoroughly covers the basics of threading and will prepare you for more advanced work with threads. Source code is provided for all the examples. No previous knowledge of threading is required, but you should be comfortable with programming in C++ at an intermediate level.The course begins by reviewing the newer features of C++ which are used in this course. If you want more thorough coverage, you can get this by taking my course "Learn Advanced Modern C++".We then cover the concepts of multithreading and learn how to launch threads in C++. We look at the problems which can occur with multiple threads and how to avoid them. C++ provides tools which allow us to work at a higher level of abstraction than system threads which share data; we cover condition variables and promises with futures. We will also look at asynchronous, lock-free and parallel programming, including atomic variables and the parallel algorithms in C++17. We will finish the course by implementing a concurrent queue and thread pool, which will bring together the material you have learnt.There are downloadable exercises for each video, with solutions, so you can check your understanding as you learn, gaining familiarity and confidence with the material. There are also some optional assignments, which are more challenging. I will be actively supporting the course. I will respond promptly if you have any questions or experience difficulties with the course content. Please feel free to use the Q&A feature or alternatively you can send me a private message.
Overview
Section 1: Introduction
Lecture 1 Introduction
Lecture 2 Lecturer Introduction
Lecture 3 Guide to Exercises and Source Code
Section 2: Overview of Modern C++ Features
Lecture 4 Modern C++ Overview Part One
Lecture 5 Modern C++ Overview Part Two
Lecture 6 Modern C++ Overview Part Three
Lecture 7 Modern C++ Overview Part Four
Lecture 8 Modern C++ Overview Part Five
Section 3: Introduction to Concurrency
Lecture 9 Concurrency Introduction
Lecture 10 Concurrency Motivation
Lecture 11 Concurrency Overview
Lecture 12 Concurrent Applications
Lecture 13 A Brief History of C++ Concurrency
Section 4: Getting Started with Threads
Lecture 14 Launching a Thread
Lecture 15 The C++ Thread Class
Lecture 16 Managing a Thread
Lecture 17 Multiple Threads
Lecture 18 Data Races
Section 5: Working with shared data
Lecture 19 Mutex Introduction
Lecture 20 Lock Guard
Lecture 21 Unique Lock
Lecture 22 Timeouts and Mutexes
Lecture 23 Shared Mutexes
Lecture 24 Shared Data Initialization
Lecture 25 Thread-local Data
Lecture 26 Lazy Initialization
Lecture 27 Deadlock
Lecture 28 Livelock
Lecture 29 Mutex Conclusion
Section 6: Thread Synchronization
Lecture 30 Condition Variables
Lecture 31 Condition Variables with Predicate
Lecture 32 Futures
Lecture 33 Promises
Lecture 34 Promises with Multiple Waiting Threads
Section 7: Atomic Types
Lecture 35 Integer Operations and Threads
Lecture 36 Atomic Types
Lecture 37 Atomic Operations
Lecture 38 Lock-free programming
Section 8: Asynchronous Programming
Lecture 39 Asynchronous Programming
Lecture 40 Packaged Task
Lecture 41 The async Function
Lecture 42 Choosing a Thread Object
Section 9: Parallelism
Lecture 43 Parallelism Overview
Lecture 44 Execution Policies
Lecture 45 Algorithms and Execution Policies
Lecture 46 New Parallel Algorithms
Lecture 47 New Parallel Algorithms Continued
Section 10: Practical Data Structures for Concurrent Programming
Lecture 48 Concurrent Data Queue Practical
Lecture 49 Thread Pools
Lecture 50 Thread Pool Implementation Practical
Section 11: Multithreading Resources
Lecture 51 Recommended Book
Lecture 52 Multithreading libraries
Programmers with a good knowledge of C++ but little or no exposure to multithreading
Homepage
https://www.udemy.com/course/learn-modern-cplusplus-concurrency/
Download ( Rapidgator )
DOWNLOAD FROM RAPIDGATOR.NET
DOWNLOAD FROM RAPIDGATOR.NET
Download (Uploadgig)
DOWNLOAD FROM UPLOADGIG.COM
DOWNLOAD FROM UPLOADGIG.COM
Download ( NitroFlare )
DOWNLOAD FROM NITROFLARE.COM
DOWNLOAD FROM NITROFLARE.COM